After my week at PDC, this afternoon I installed Windows 7 on a spare machine. While I have noticed a few bugs, it generally appears to be very good – if not very similar to Windows Vista.
During the 2nd keynote I noticed they have changed UAC, so I decided to investigate what they have done. When Vista was launched, Microsoft spent a lot of time and effort in trying to educate developers into understanding why its important for people not to run as administrator. Personally, I think it worked – for a large part of applications, you can run without needing to be an administrator. UAC has had a direct result on making improved more secure software.
However, things are different with Windows 7. By default (PDC build 6801), only certain actions will cause the UAC dialog to appear. For Administrators, you will not be notified via UAC when you make a change to the Windows settings, but you still will when a program installs. However, during the install the user you create is set as an Administrator. This means it will apply to a large portion of the Windows user base as they will all be running as Administrator.
If your interested, the new control panel dialog for UAC looks like this with 4 different levels based on the amount of security you wish to have:
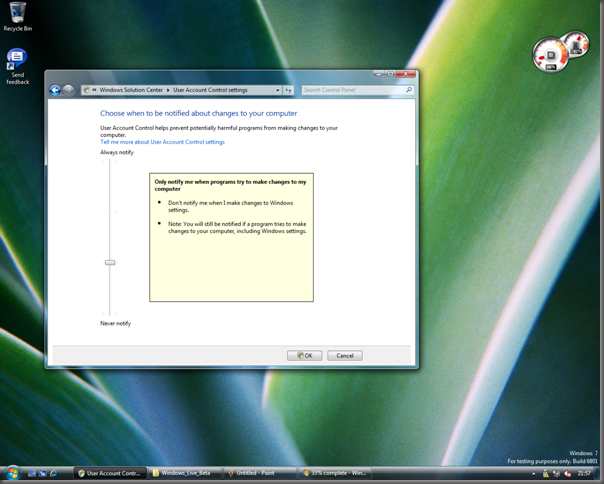
The result of this default level is that Windows won’t cause UAC, but other 3rd party applications will (until hackers figure out to work around this). The users perception is now that UAC is only annoying when using other people’s programs, but not Windows. Marketing are happy while the end user is left confused and vulnerable as they are unaware of when UAC should be displayed with the core message of protecting you and your system being lost.
My Mother recently got a new HP laptop with Vista pre-installed which she happily configured from new, hence running as Administrator at the time. She had been hit with viruses and malware before with XP, but Vista the message to stay secure was simple. ‘If you see this a dialog with the darken background, call me or click cancel’. As a result, the laptop still works. No settings has been changed, no random applications installed and I’ve been asked once during the last 6 months why it has appeared.
With Windows 7, the message is more like ‘You can play around with all the Windows settings without being prompted, however you might be if other things change it, as a results it’s just best if you don’t touch anything.’
Take changing the time for example, by default a dialog will not appear when you click the ‘Change date and time…’, however it does have the UAC shield, which according to Microsoft means you should expect to see a UAC dialog (hence causing more confusion). Under the covers, Windows is silently evaluating the process, as a result it does have UAC permissions granted by Windows.
When applications are installing, you will see the UAC screen, however it no longer has the darkened screen. Why this makes for a more fluent experience, the dialog is just that – a dialog. It means I have to spend even less time reading and thinking about it hence I will just click Yes. At least when the screen changed, people (mainly me) woke up and looked at what was happening.
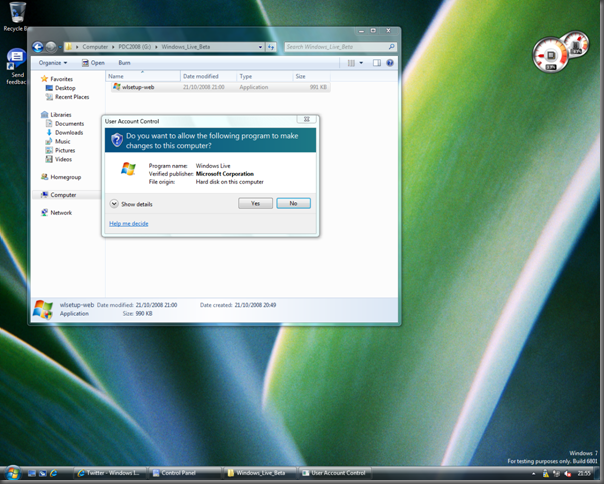
One more serious note – by default – from the default level to off doesn’t cause a confirmation UAC dialog!
To me, this is the extremely bad decision. UAC was designed to be annoying for a reason!! It was designed to make users click before confirming their action. Please Microsoft, don’t change this. Please don’t make us go back to the dark days of having to run as Admin without UAC for all of our applications to work.